Java Input and Output
In Java, all the input/output are doing using the standard built-in classes and methods. Most of the input and output classes are available in the package java.io. Java has several IO packages, a programmer can choose the one that matches his/her requirements.
IO Streams
A stream is a sequence of characters or bytes used for program input or output. Java provides various input and output stream classes with the java.io API.
The commonly used IO objects are:
- System.in (Standard input stream)
- System.out (Standard output stream for any message)
- System.err (Standard output stream for error messages)
Output stream
Used to output data generated by the program. Computer screens are typically used for standard output streams and are represented as System.out.
Hierarchy
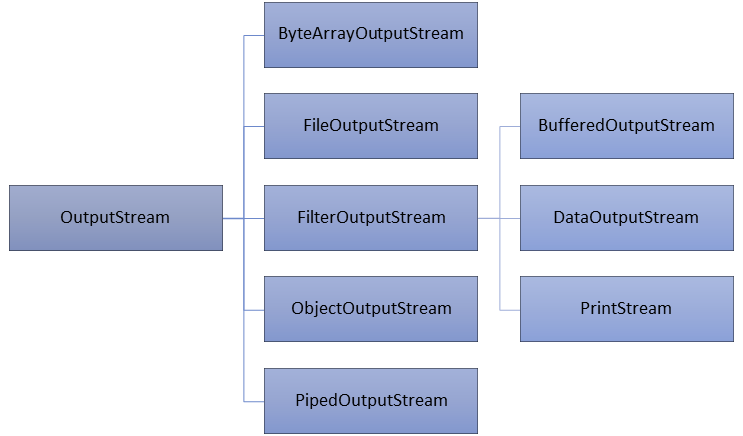
Java output stream hierarchy
Example
public class Output { public static void main(String[] args) { // Without new line System.out.print("Line without new line"); // Move to new line System.out.print("\n"); // With new line System.out.println("Line with new line"); // Using printf System.out.printf("My name is %s, and i, am %d year old", "John", 24); } }
Error stream
Used to output error data generated by the program. Computer screens are usually used for standard error streams and are represented as System.err.
public class ErrorOutput { public static void main(String[] args) { // Without new line System.err.print("Error line without new line"); // Move to new line System.err.print("\n"); // With new line System.err.println("Error line with new line"); // Using printf System.err.printf("Error : %s, at line no %d", "NullPointerException", 10); } }
See for the explanation – Java program to print to console
Input stream
Used to give data to the user’s program, typically the keyboard is used as a standard input stream, represented as System.in.
Hierarchy
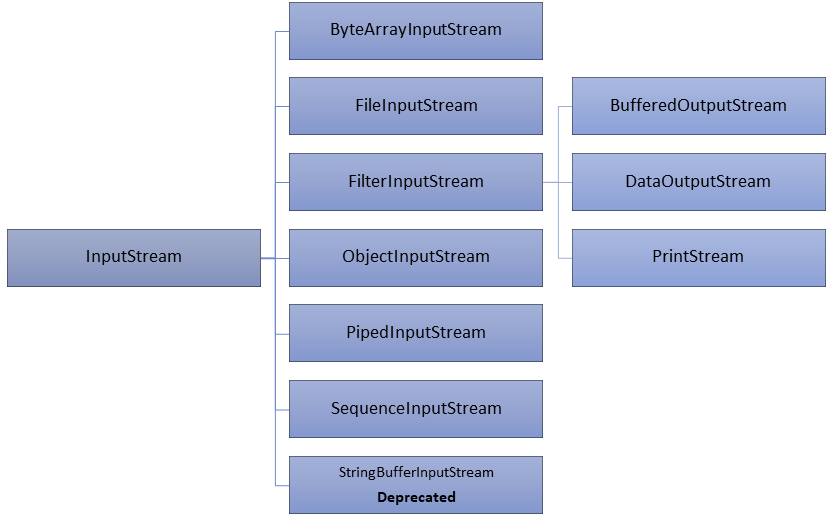
Java input stream hierarchy
There are several ways to take input from the user. We will look at two of them –
- BufferedReader
- Scanner
BufferedReader example
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; public class BufferedReaderExample { public static void main(String[] args) throws IOException { BufferedReader reader = new BufferedReader(new InputStreamReader(System.in)); System.out.println("Enter your first name : "); String firstName = reader.readLine(); System.out.println("Enter your last name : "); String lastName = reader.readLine(); System.out.printf("Your full name is : %s %s\n", firstName, lastName); } }
Scanner example
import java.util.Scanner; public class Main { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("Enter your first name : "); String firstName = scanner.nextLine(); System.out.println("Enter your last name : "); String lastName = scanner.nextLine(); System.out.printf("Your full name is : %s %s\n", firstName, lastName); } }